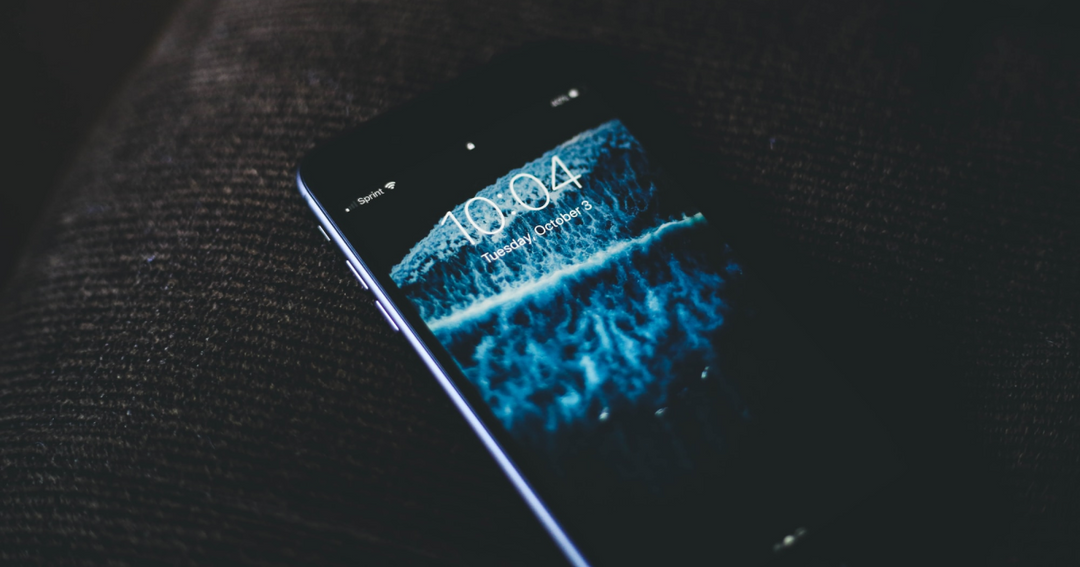
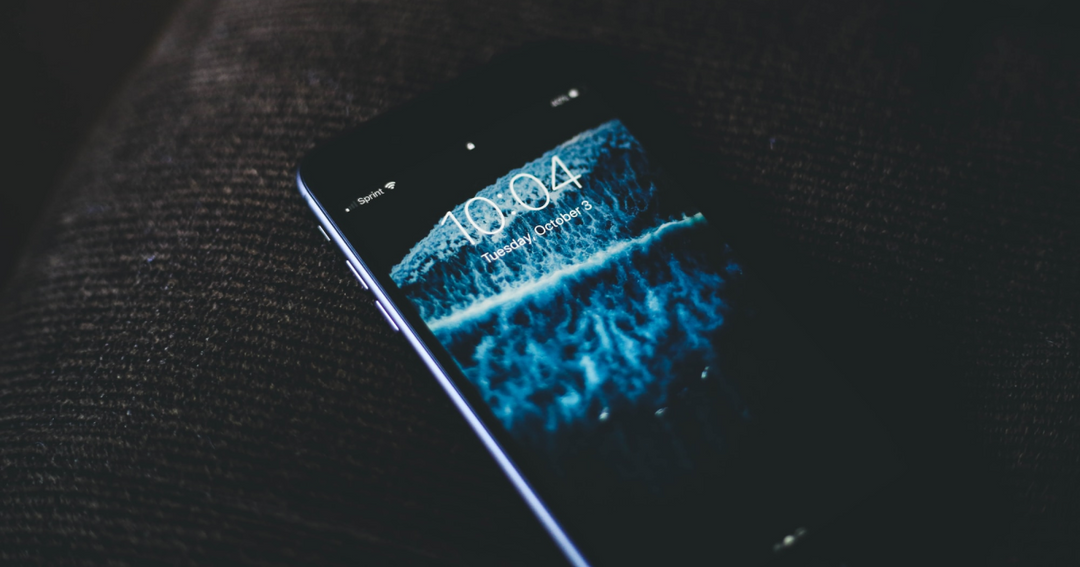
· By Codomo Singapore
I cracked 40,000 passwords with Python. Yours might have been one of them
Remember the good old days when you were passing love notes to your crush across the classroom? Chances are you’ve had to pass that note to your friend > another friend > and another friend before it reaches your crush. And friends are the worst; you can’t trust them with your secret message. In response, you probably established some kind of code between you and your crush beforehand. The message makes sense to both of you but appears as gibberish to the people in between. That’s what we called encryption.
🚨 JARGON ALERT:
Encryption and hashing are similar; they make words become gibberish. The difference is encryption is reversible, while hashing is (almost) irreversible. For passwords, we use hashing.
HOW PASSWORDS ARE STORED IN COMPANIES
- Plain text (Can you hear me shaking my head?)
- Hashed passwords
- Salted hashed passwords
Responsible companies hash your passwords. They take the password you type into their sign-up page, make it gibberish, then store those gibberish words into their database. In the event a hacker flirts with your database administrator and gains access to the database, all he’ll see is just the gibberish stuff. They can’t just copy your gibberish password and paste it into the login page because the algorithm will make a gibberish out of the gibberish word. I’ll let that sink in.
Even more responsible companies salt your passwords. Meaning, they “add random characters at random position” to your password entries before sending it for hashing. For example, you enter a shitty password — “Password”. With salting, the algorithm probably adds a few characters to it till it becomes something like this “xyzPassword123”. “Password” is in the dictionary, however, “xyzPassword123” is not. This makes guessing the actual password way tougher ☝️.
SETTING EXPECTATIONS
In the next part of this article, I’m going to show you how hackers “decrypt” hashed password to the actual word (Well, they don’t actually decrypt, they guess). Then, I’m going to show you how it’s done in Python.
Before I proceed any further, I would like to point out that the purpose of this article is to show you the big picture; I will avoid being technical here. If I say anything technical, you will hear me saying sorry. Yes, I have oversimplified many things here. Cool? Let’s go.
HOW DICTIONARY ATTACKS WORK
In short, a dictionary attack (sorry!) is the cracking of a password, based on the words that appear in the dictionary (durh..). There are 3 steps to a dictionary attack.
- ACCESS to the (hashed) password list
- HASH all the words found in the English dictionary
- COMPARE the (hashed) English words with the (hashed) passwords
STAGE 1: ACCESS
Let me give you an example. Let’s say I flirted with a database administrator of a company and managed to gain access to the following 3 hashed passwords:
- 5f4dcc3b5aa765d61d8327deb882cf99
- 9b4609b17fea63f3f3f067fc2f465c6e
- 24ebcd0fd5d6b86649fb187d75f80ad0
STAGE 2: HASH
Using programming, I hashed all of the 350,000+ English words. I will use a hashing method called “md5” (sorry!). There are many hashing methods — MD5, SHA1, SHA2, SHA3 (sorry, sorry, sorry, sorry) etc.
STAGE 3: COMPARE
I then comb through all of the 370,000+ (hashed) English words. If I find a match, bingo! That’s the password.
Still confused? I have created a 20 second blockbuster movie below for you to see how it works:
DICTIONARY ATTACKS (Using Python)
>>> You can download my entire python code here. <<<
I started with 2 text files: english.txt and 1MillionPassword.txt.
- english.txt is a text file containing english words
- 1MillionPassword.txt is a text file containing 1 million passwords that humans have created in the real world.
📰 Breaking news
In Dec 2009, a company named Rockyou experienced a data breach where 32 million user accounts were exposed. To make matter worse, 14 million passwords were exposed, IN PLAIN TEXT!! Yes, the 1MillionPassword.txt is derived from this 14 million.
ASSUMPTIONS
For the purpose of this article, I’m going to hash the rockyou passwords with md5 hashing (Meaning, we’re assuming that rockyou did hash the 14 million passwords before the hack). I mean, what’s there to crack if the password is revealed to us in plain text… ¯\_(ツ)_/¯
PYTHON LIBRARIES | SPECS OF MY MACHINE
There’s a python library called hashlib where you can convert text to the hashed characters. I’ve also used concurrent.futures library to run parallel tasks to make my code run faster. Yes, I am slave-driving my 4 CPU cores. I’m running on a Macbook with a 3.1 GHz Intel Core i7 CPU, and Intel Iris Graphics 6100 1536 MB GPU.
CREATING THE PYTHON SCRIPT
I created 2 python files: createHash.py and crackPassword.py.
- createHash.py — Hash the 370,000 words in english.txt and 1MillionPassword.txt using md5 (sorry!).
- crackPassword.py — Splits the 1 million passwords into 4 lists. Each CPU core will take a list and make a comparison between the (hashed) English word and the (hashed) password.
THE RESULT
Summary:
CPU 1: 26826/250000 password has been cracked. 72.49 minutes elapsed.
CPU 2: 8138/249999 password has been cracked. 74.19 minutes elapsed.
CPU 3: 4671/249999 password has been cracked. 74.38 minutes elapsed.
CPU 4: 653/249999 password has been cracked. 74.59 minutes elapsed.
Total passwords cracked: 40,288 in a little more than an hour
FAQ
You: “All right, Sherlock; then how do you bypass the maximum login attempts allowed? Hm? You can’t just brute force over there, bro.”
Me: “Of course. Hackers are probably not interested in accessing the account they’ve just hacked. They are probably more interested in the higher valued accounts, like your email address. Why? Because if they managed to compromise your email, they can try a ‘forget password’ on all of your other accounts. They can start hacking into your Facebook account and start liking your crush’s photos 5 years ago. No amount of justification can save you from that embarrassment, you ‘creep’.”
You: “Wait. How would they know my email’s password? They are not the same.”
Me: “People reuse passwords. That’s the problem.”
You: I’m still not convinced. I don’t think there are a lot of people who reuse the exact same password for their email.
Me: Well, maybe. I don’t know the statistics of how many people reuse their email password on other accounts. The problem is that if a hacker manages to gain access to one or two emails, he can send a malicious email to the victims’ friends. People tend to click at an email that arrives from a friendly source. The process will go something like this.
‘Victim’ sends an email > A few friends open it > Malicious software gets downloaded > Their computers gets compromised > Starts spreading to other friends.
It’s almost like a virus.
You: What about 2-step verification?
Me: Thank goodness we have this. Wait, did you turn on your 2-step verification?
CLOSING THOUGHTS
Protecting ourself from cyber crime is like buying insurance. People don’t subscribe to it until shit hits the fan. I have 6 tips for you to protect yourself and your loved ones.
- Secure your main email address to the highest security. Use a strong password, don’t reuse it, and turn on 2FA.
- Don’t use a password that can be found in the dictionary. I just showed you how easy it is to crack. “Password” and “P@$$w0rd” are equally vulnerable.
- Use passphrases, not complicated passwords. Meaning, don’t use Tr0ub4dor&3, use something like correct-horse-battery-staple; it’s longer and more memorable. Yes, I stole this example from xkcd.
- Invest in a password manager to diversify your password; I use 1Password for its security and beautiful UI. It costs $2.99/month (that’s cheaper than a cup of latte from Starbucks).
- If you have children, consider buying a “Potato Pirates: Enter the Spudnet” for them. It’s a board game that teaches cybersecurity.
- If you want to know more about the different types of cyber attacks, you can check out this article.